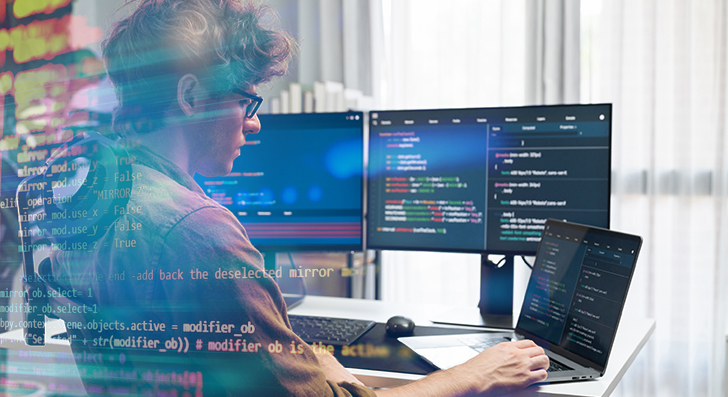
Scalability indicates your application can manage growth—more people, far more info, and much more traffic—without breaking. To be a developer, constructing with scalability in your mind saves time and worry later on. Here’s a transparent and useful manual to help you start out by Gustavo Woltmann.
Structure for Scalability from the Start
Scalability isn't a thing you bolt on afterwards—it should be aspect of the strategy from the start. Numerous purposes fall short when they increase quickly because the initial style can’t handle the extra load. Being a developer, you should Believe early regarding how your process will behave under pressure.
Start out by creating your architecture to generally be adaptable. Steer clear of monolithic codebases wherever every thing is tightly related. Rather, use modular style and design or microservices. These patterns split your app into more compact, independent pieces. Every single module or service can scale By itself without influencing the whole process.
Also, take into consideration your database from day just one. Will it require to manage one million customers or maybe 100? Choose the proper type—relational or NoSQL—depending on how your knowledge will mature. Plan for sharding, indexing, and backups early, even if you don’t need to have them nonetheless.
An additional critical issue is in order to avoid hardcoding assumptions. Don’t publish code that only will work less than present-day conditions. Consider what would take place If the person foundation doubled tomorrow. Would your application crash? Would the databases decelerate?
Use style and design styles that guidance scaling, like information queues or celebration-pushed devices. These enable your app deal with much more requests without having acquiring overloaded.
If you Create with scalability in mind, you're not just preparing for success—you happen to be minimizing potential head aches. A effectively-planned procedure is less complicated to keep up, adapt, and expand. It’s far better to get ready early than to rebuild later on.
Use the correct Database
Deciding on the suitable database is really a key Element of making scalable programs. Not all databases are designed precisely the same, and using the Incorrect you can sluggish you down or perhaps cause failures as your application grows.
Commence by comprehending your data. Is it highly structured, like rows in a very table? If yes, a relational databases like PostgreSQL or MySQL is an effective in good shape. These are typically robust with relationships, transactions, and regularity. Additionally they support scaling tactics like study replicas, indexing, and partitioning to take care of a lot more traffic and knowledge.
If your facts is more adaptable—like consumer exercise logs, solution catalogs, or files—think about a NoSQL possibility like MongoDB, Cassandra, or DynamoDB. NoSQL databases are far better at managing massive volumes of unstructured or semi-structured information and will scale horizontally far more easily.
Also, contemplate your browse and compose styles. Are you currently undertaking lots of reads with less writes? Use caching and browse replicas. Are you presently handling a hefty produce load? Consider databases that could tackle higher publish throughput, or maybe event-primarily based knowledge storage devices like Apache Kafka (for short term details streams).
It’s also intelligent to Feel forward. You might not have to have advanced scaling functions now, but picking a databases that supports them suggests you received’t want to change later on.
Use indexing to speed up queries. Keep away from unwanted joins. Normalize or denormalize your details depending on your access patterns. And usually check databases effectiveness when you improve.
To put it briefly, the ideal databases relies on your application’s composition, velocity requires, And exactly how you anticipate it to expand. Get time to choose properly—it’ll conserve lots of difficulty later.
Improve Code and Queries
Rapid code is essential to scalability. As your application grows, every single compact hold off adds up. Badly created code or unoptimized queries can slow down general performance and overload your procedure. That’s why it’s essential to Create productive logic from the start.
Get started by writing clean up, uncomplicated code. Keep away from repeating logic and remove anything avoidable. Don’t select the most sophisticated Answer if a straightforward one particular operates. Keep your functions shorter, centered, and easy to check. Use profiling resources to find bottlenecks—destinations in which your code takes far too extended to operate or employs an excessive amount memory.
Subsequent, evaluate your database queries. These normally sluggish things down a lot more than the code itself. Ensure that Each and every question only asks for the data you truly need to have. Steer clear of Pick out *, which fetches every thing, and as a substitute choose precise fields. Use indexes to speed up lookups. And keep away from accomplishing too many joins, In particular across huge tables.
In the event you detect exactly the same knowledge being requested time and again, use caching. Retailer the final results temporarily making use of applications like Redis or Memcached which means you don’t really have to repeat high-priced functions.
Also, batch your databases operations once you can. In place of updating a row one after the other, update them in groups. This cuts down on overhead and helps make your application extra effective.
Remember to take a look at with significant datasets. Code and queries that get the job done fine with 100 information could crash when they have to deal with 1 million.
Briefly, scalable applications are speedy applications. Keep the code limited, your queries lean, and use caching when required. These actions enable your software continue to be sleek and responsive, at the same time as the load improves.
Leverage Load Balancing and Caching
As your application grows, it's got to take care of more users and much more site visitors. If every little thing goes by means of a person server, it will eventually quickly turn into a bottleneck. That’s where by load balancing and caching are available. Both of these instruments support maintain your app quick, stable, and scalable.
Load balancing spreads incoming traffic throughout various servers. Rather than 1 server doing all the work, the load balancer routes buyers to unique servers based upon availability. What this means is no single server gets overloaded. If a person server goes down, the load balancer can send out traffic to the Other people. Applications like Nginx, HAProxy, or cloud-based methods from AWS and Google Cloud make this very easy to create.
Caching is about storing information quickly so it could be reused swiftly. When users ask for the identical info all over again—like a product web page or simply a profile—you don’t ought to fetch it with the database when. It is possible to serve it within the cache.
There are 2 frequent varieties of caching:
one. Server-facet caching (like Redis or Memcached) outlets knowledge in memory for quickly obtain.
2. Customer-side caching (like browser caching or CDN caching) merchants static files near the user.
Caching lessens database load, enhances velocity, and can make your application far more efficient.
Use caching for things that don’t change typically. And usually make certain your cache is up-to-date when information does improve.
In a nutshell, load balancing and caching are simple but highly effective tools. Jointly, they assist your app tackle a lot more buyers, remain rapid, and recover from complications. If you plan to increase, you need both of those.
Use Cloud and Container Applications
To create scalable purposes, you will need instruments that permit your app increase conveniently. That’s exactly where cloud platforms and containers can be found in. They offer you adaptability, website lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Enable you to lease servers and expert services as you would like them. You don’t need to acquire hardware or guess foreseeable future ability. When website traffic improves, you can add far more methods with just a couple clicks or mechanically working with vehicle-scaling. When targeted visitors drops, you could scale down to economize.
These platforms also give products and services like managed databases, storage, load balancing, and stability instruments. You may center on setting up your application in place of controlling infrastructure.
Containers are One more vital Resource. A container deals your app and every thing it must operate—code, libraries, configurations—into one particular unit. This makes it easy to maneuver your application among environments, from your notebook to your cloud, with no surprises. Docker is the most well-liked Device for this.
When your application works by using a number of containers, instruments like Kubernetes enable you to handle them. Kubernetes handles deployment, scaling, and Restoration. If one particular element of your application crashes, it restarts it instantly.
Containers also make it very easy to independent aspects of your application into solutions. You could update or scale areas independently, that's great for effectiveness and reliability.
To put it briefly, making use of cloud and container tools suggests you are able to scale rapid, deploy very easily, and Get better swiftly when complications take place. If you want your app to mature without having restrictions, begin working with these equipment early. They help you save time, lessen hazard, and enable you to continue to be focused on creating, not correcting.
Keep track of Almost everything
For those who don’t check your software, you received’t know when issues go Mistaken. Checking helps you see how your app is undertaking, location troubles early, and make improved decisions as your app grows. It’s a essential Element of developing scalable programs.
Start out by monitoring basic metrics like CPU usage, memory, disk Room, and reaction time. These inform you how your servers and products and services are doing. Resources like Prometheus, Grafana, Datadog, or New Relic can assist you accumulate and visualize this details.
Don’t just monitor your servers—keep track of your app also. Regulate how much time it takes for customers to load pages, how often problems transpire, and wherever they manifest. Logging applications like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly will let you see what’s going on within your code.
Arrange alerts for vital problems. For example, In case your response time goes above a Restrict or a company goes down, you'll want to get notified quickly. This will help you resolve concerns quick, frequently before users even see.
Checking can be beneficial whenever you make modifications. If you deploy a completely new element and see a spike in errors or slowdowns, you could roll it back again just before it leads to real problems.
As your app grows, traffic and details maximize. Devoid of monitoring, you’ll pass up indications of difficulty right until it’s way too late. But with the correct applications in position, you stay on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not almost spotting failures—it’s about comprehension your method and ensuring that it works very well, even under pressure.
Closing Thoughts
Scalability isn’t only for large businesses. Even smaller apps need to have a strong foundation. By building very carefully, optimizing sensibly, and using the suitable tools, you may build apps that mature easily devoid of breaking under pressure. Commence smaller, Believe massive, and Establish wise.